eSentry
A full-stack eBay monitoring platform that evolved from a university project into a fully deployed product. Underwent a complete refactoring from Angular.js to Next.js with a Node.js backend to improve performance and scalability.
Technologies Used
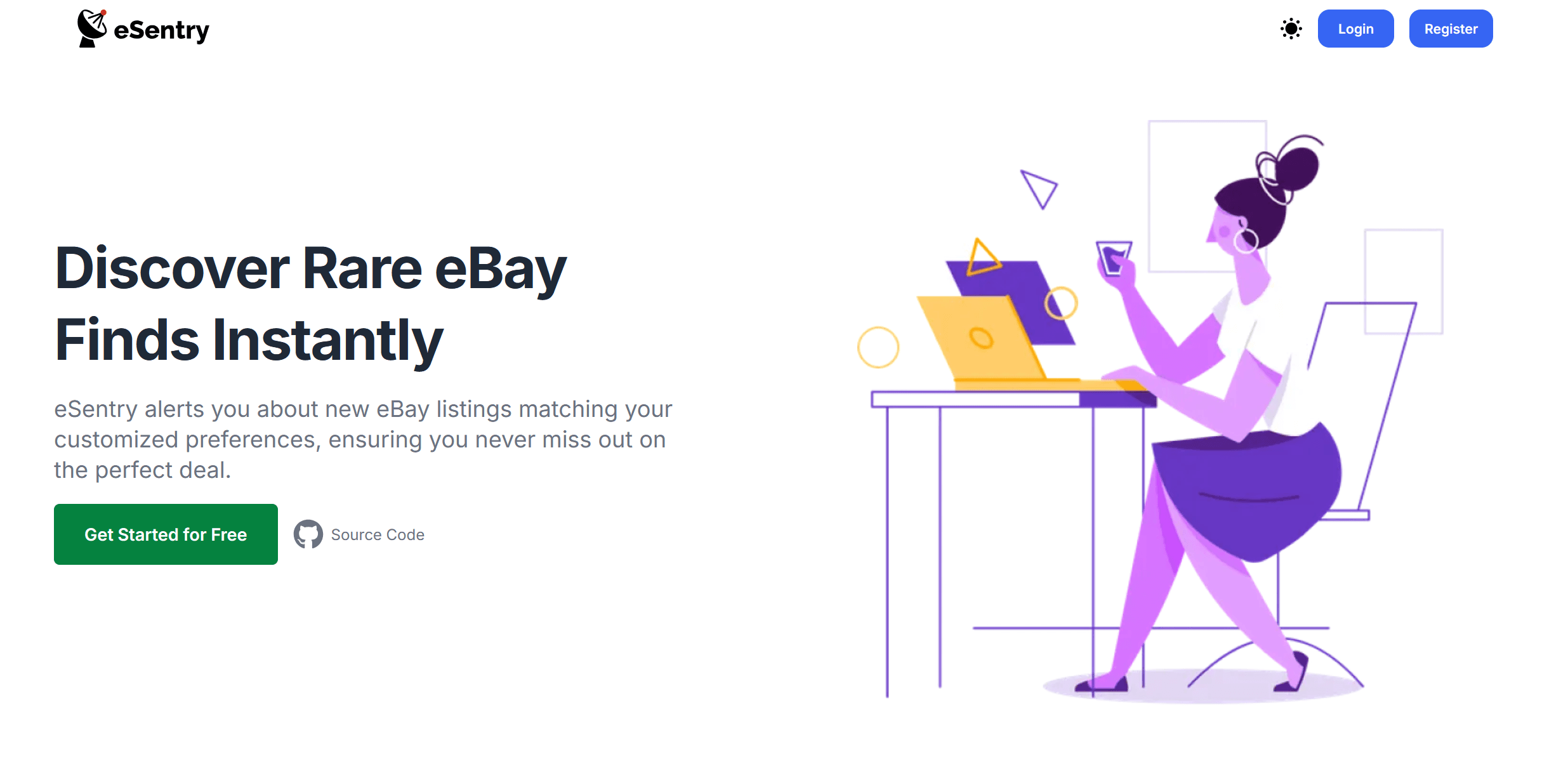
Project Overview
eSentry is a real-time monitoring platform that helps users track rare or price-sensitive listings on eBay. The application evolved from a simple university project into a fully-featured product with a modern tech stack and enhanced user experience.
From University Project to Production
eSentry began as a university final project with basic functionality that allowed users to create an eBay monitor that would notify them via Telegram when items matching their criteria were listed. The initial implementation used Axios for web scraping eBay search results and a Telegram bot API for notifications, as these tools provided the most accessible path to a working prototype.
After completing the course, I recognized the potential to transform this rudimentary project into a polished product. I set out to rebuild it using industry-standard development practices while significantly improving the user experience. The goal was to create something that wasn't just functional but also intuitive and accessible to users with varying levels of technical knowledge.
eSentry addresses a specific gap in the market: helping buyers find limited-supply items or products listed at unexpectedly competitive prices. Many sellers on eBay list items without knowing their true market value or simply want to clear inventory quickly, creating opportunities for savvy buyers to find exceptional deals—if they can be first to discover these listings.
While eBay does offer an inventory digest via email, it lacks the real-time alerts that eSentry provides. This critical difference means eSentry users can react immediately to new listings, giving them a significant advantage in securing high-demand items or special deals before other buyers. Identifying this market gap motivated me to transform the initial concept into a comprehensive, user-friendly solution that delivers genuine value to online shoppers.
Technical Architecture
System Architecture Overview
eSentry utilizes a modern full-stack architecture with clear separation between frontend and backend services. The application is built with a Next.js frontend communicating with a Node.js backend API, both interacting with a shared PostgreSQL database through Prisma ORM.
I designed the system with modularity in mind, allowing each component to focus on its specific responsibility while maintaining clean communication patterns between services. The frontend and backend communicate via RESTful API endpoints, enabling structured CRUD operations for user accounts, monitors, and all other application features.
Data Layer & Shared Schema
For database management, I created a shared Prisma Schema npm package that serves as a single source of truth for all database models and their relations. This approach makes database changes and migrations significantly easier—I simply update the package and both frontend and backend automatically receive the updated schema.
The Prisma ORM abstracts away complex SQL queries in favor of a data-centered JSON approach, making database interactions more intuitive and maintainable. Here's an example of how monitor creation works with Prisma:
1// Create the eSentry monitor from the user request data object
2const monitor = await prisma.monitor.create({
3 data: {
4 userId,
5 keywords: userReq.keywords,
6 excludedKeywords: userReq.excludedKeywords || [],
7 minPrice: userReq.minPrice,
8 maxPrice: userReqmaxPrice,
9 conditions: userReq.conditions || [],
10 sellers: userReq.sellers || [],
11 status: 'inactive',
12 },
13});
This code structure allows me to focus on the data relationships rather than writing complex SQL queries, significantly improving development speed and reducing potential errors.
Authentication & Security
The frontend implements Auth.js to provide a streamlined authentication experience with OAuth providers (Google and GitHub). This not only simplifies the login process for users but also enhances security by leveraging established authentication providers.
Security is implemented in layers throughout the application:
- Auth.js protects frontend API routes before requests even reach the backend
- Backend middleware authenticates requests again by verifying the user ID from frontend requests
- Rate limiting using Redis caching prevents API overload by tracking how many calls each user makes
This multi-layered approach ensures that only authenticated users can access sensitive functionality and prevents potential abuse of the system.
Monitoring & Queue System
The heart of eSentry's monitoring capability is built around an efficient job queue system. When a user activates a monitor, their request enters a BullMQ queue waiting for processing. These jobs run at fixed intervals to check for new eBay listings.
The monitoring process works by:
- Pulling data from the eBay Search API using the user's specified monitor parameters
- Caching search results for one interval, creating a comparison point
- Comparing new search results against the cached data to identify new listings
- Triggering email notifications through Amazon SES when new matches are found
This approach ensures reliable monitoring while maintaining reasonable load on both the backend server and eBay's API.
Deployment & Infrastructure
The backend is fully containerized using Docker, which provides consistent environments across development and production. The Docker setup includes not just the API service but also PostgreSQL and Redis services, creating a complete self-contained system.
For hosting, I deployed the backend container to a DigitalOcean VPS running Ubuntu. The application uses a domain structure that separates concerns:
- Frontend application:
esentry.dev
- Backend API:
api.esentry.dev
This separation with HTTPS implementation ensures secure communication between all system components. The containerized approach also makes scaling and updating straightforward, as I can deploy new versions with minimal downtime and consistent behavior.